Hello,
I need to calculate the total cost of products based on certain charges related to international vendors. These charges include:
- Container Fee (for the space the product occupies in a shipping container),
- Customs Duty (a percentage charge on the base price of the product),
- Handling Charge (additional fees for handling the product).
I created those fields on the res.partner model for international suppliers. I want to use these values in the product.template model to compute the total cost of the product (without profit margin).
In the res.partner model I have created those fields:
- x_container_fee (Type: Monetary): This field stores the container fee per square meter (or unit of space) that an international vendor charges (vary depending on country).
- x_customs_duty (Type: Float): This field represents the customs duty rate (as a percentage) that is applied to products from this international vendor.
- x_handling_charge (Type: Monetary): This field stores any additional handling charge imposed by the vendor.
- x_vendor_type (Type: Selection): This field indicates whether the vendor is local or international. Only for international vendors do the additional charges (customs, container fee, and handling charge) apply.

In the product.template model:
- x_container_space (Type: Float): This field represents the space (in square meters) the product occupies in the shipping container.
- x_total_cost (Type: Monetary): This field stores the final total cost of the product, which includes the base price and the additional charges from the international vendor (without the profit margin).

Calculation Process:
For each product, I want to compute the total cost as follows:
- Base Price: Start with the product's base price (either list price or cost price).
- International Supplier Charges: If the vendor is marked as international, I want to apply:
- Customs Duty: Calculate the customs duty as a percentage.
- Container Fee: I will insert on the each product base on the area it will occupy in the container.
-
Handling Charge: Add any fixed handling charges from the vendor.
- Final Total Cost: Sum up these values to get the total cost of the product without including the profit margin.
Code I have use:
from odoo import api, fields, models
class ProductTemplate(models.Model):
_inherit = "product.template"
# Link to the vendor (res.partner) model
vendor_id = fields.Many2one('res.partner', string='Vendor', help="Reference to the vendor from res.partner.")
# Related fields from the vendor
x_container_fee = fields.Monetary(related='vendor_id.x_container_fee', string='Container Fee', readonly=True)
x_customs_duty = fields.Float(related='vendor_id.x_customs_duty', string='Customs Duty', readonly=True)
x_handling_charge = fields.Monetary(related='vendor_id.x_handling_charge', string='Handling Charge', readonly=True)
# Space occupied by the product in the container (in square meters or units)
x_container_space = fields.Float(string='Container Space (m²)', help="Space occupied by the product in square meters.")
# Field to store the calculated total cost
x_total_cost = fields.Monetary(string='Total Cost', compute='_compute_total_cost', store=True, help="Total cost of the product without profit margin.")
@api.depends('list_price', 'x_container_fee', 'x_customs_duty', 'x_handling_charge', 'x_container_space')
def _compute_total_cost(self):
for product in self:
# Base price paid to the vendor (list_price or cost price)
base_price = product.list_price
# Initialize additional charges
total_customs_duty = 0
total_container_fee = 0
total_handling_charge = 0
# Check if the vendor is assigned and marked as international
if product.vendor_id and product.vendor_id.x_vendor_type == 'international':
# Calculate customs duty (percentage of the base price)
customs_duty_rate = product.x_customs_duty or 0
total_customs_duty = base_price * customs_duty_rate / 100
# Calculate container fee based on the space occupied
container_fee_per_sqft = product.x_container_fee or 0
space_occupied = product.x_container_space or 1 # Default to 1 if not specified
total_container_fee = container_fee_per_sqft * space_occupied
# Add container handling charge
total_handling_charge = product.x_handling_charge or 0
# Calculate total cost (without profit margin)
product.x_total_cost = base_price + total_customs_duty + total_container_fee + total_handling_charge
When I created the total cost and put the Python code I need to put the Dependencies to which I am having the error:
Unknown field “x_container_fee” in dependency “x_container_fee”
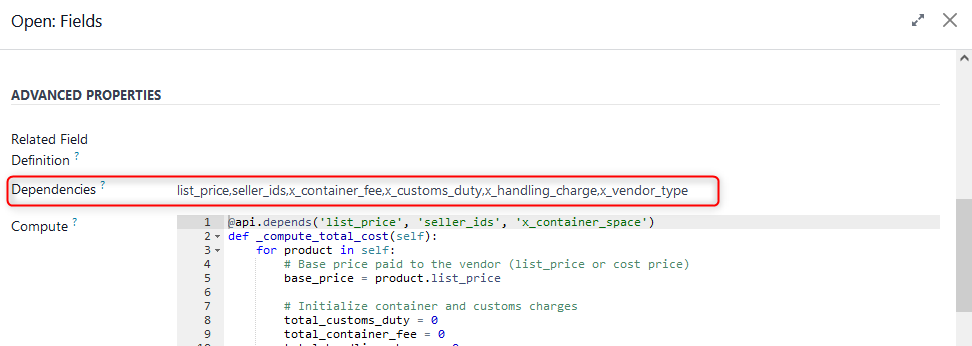